JUPYTER NOTEBOOK CHEAT SHEET Learn PYTHON from experts at Keyboard Shortcuts Jupyter Notebook is an open-source web application that allows you to create and share documents that contain live code, equations, visualizations and narrative text. It is used for data cleaning and transformation, numerical simulation, statistical. To help you overcome the “valley of desperation”, I created a series of Python cheat sheets—this one being the first and most basic one. This cheat sheet is for beginners in the Python programming language. It explains everything you need to know about Python keywords. Download and pin it to your wall until you feel confident using all.
Python selenium commands cheat sheet
Frequently used python selenium commands – Cheat Sheet
To import webdriver module in python use below import statement
Driver setup:
Firefox:
firefoxdriver = webdriver.Firefox(executable_path=”Path to Firefox driver”)
To download: Visit GitHub
Chrome:
chromedriver = webdriver.Chrome(executable_path=”Path to Chrome driver”)
To download: Visit Here
Internet Explorer:
iedriver = webdriver.IE(executable_path=”Path To IEDriverServer.exe”)
To download: Visit Here
Edge:
edgedriver = webdriver.Edge(executable_path=”Path To MicrosoftWebDriver.exe”)
To download: Visit Here
Opera:
operadriver = webdriver.Opera(executable_path=”Path To operadriver”)
To download: visit GitHub
Safari:
SafariDriver now requires manual installation of the extension prior to automation
Browser Arguments:
–headless
To open browser in headless mode. Works in both Chrome and Firefox browser
–start-maximized
To start browser maximized to screen. Requires only for Chrome browser. Firefox by default starts maximized
–incognito
To open private chrome browser
–disable-notifications
To disable notifications, works Only in Chrome browser
Example:
or

To Auto Download in Chrome:
To Auto Download in Firefox:
We can add any MIME types in the list. MIME for few types of files are given below.
- Text File (.txt) – text/plain
- PDF File (.pdf) – application/pdf
- CSV File (.csv) – text/csv or “application/csv”
- MS Excel File (.xlsx) – application/vnd.openxmlformats-officedocument.spreadsheetml.sheet or application/vnd.ms-excel
- MS word File (.docx) – application/vnd.openxmlformats-officedocument.wordprocessingml.document
Zip file (.zip) – application/zip
Note:
The value of browser.download.folderList can be set to either 0, 1, or 2.
0 – Files will be downloaded on the user’s desktop.
1 – Files will be downloaded in the Downloads folder.
2 – Files will be stored on the location specified for the most recent download
Disable notifications in Firefox
firefoxOptions.set_preference(“dom.webnotifications.serviceworker.enabled”, false);
firefoxOptions.set_preference(“dom.webnotifications.enabled”, false);
Open specific Firefox browser using Binary:
Open specific Chrome browser using Binary:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
options = Options()
options.binary_location = “”
driver = webdriver.Chrome(chrome_options=options, executable_path=””)
driver.get(‘http://google.com/’)
Read Browser Details:
driver.title
driver.window_handles
driver.current_window_handles
driver.current_url
driver.page_source
The new Citrix Workspace app (formerly known as Citrix Receiver) provides a great user experience - a secure, contextual, and unified workspace - on any device. It gives you instant access to all your SaaS and web apps, your mobile and virtual apps, files, and desktops from an easy-to-use, all-in-one interface powered by Citrix Workspace services. Using your mobile and virtualized applications. Download Citrix Workspace App, Citrix ADC and all other Citrix workspace and networking products. Receive version updates, utilities and detailed tech information. Citrix Workspace app is the easy-to-install client software that provides seamless, secure access to everything you need to get work done. With this free download, you easily and securely get instant access to all applications, desktops and data from any device, including smartphones, tablets, PCs and Macs.
Go to a specified URL:
driver.get(“http://google.com”)
driver.back()
driver.forward()
driver.refresh()
Locating Elements:
driver.find_element_by_ – To find the first element matching the given locator argument. Returns a WebElement
driver.find_elements_by_ – To find all elements matching the given locator argument. Returns a list of WebElement
By ID
<input id=”q” type=”text” />
element = driver.find_element_by_id(“q”)
By Name
<input id=”q” name=”search” type=”text” />
element = driver.find_element_by_name(“search”)
By Class Name
<div class=”username” style=”display: block;”>…</div>
element = driver.find_element_by_class_name(“username”)
By Tag Name
<div class=”username” style=”display: block;”>…</div>
element = driver.find_element_by_tag_name(“div”)
By Link Text
<a href=”#”>Refresh</a>
element = driver.find_element_by_link_text(“Refresh”)
By Partial Link Text
<a href=”#”>Refresh Here</a>
element = driver.find_element_by_partial_link_text(“Refresh”)
By XPath
<form id=”testform” action=”submit” method=”get”>
Username: <input type=”text” />
Password: <input type=”password” />
</form>
element = driver.find_element_by_xpath(“//form[@id=’testform’]/input[1]”)
By CSS Selector
<form id=”testform” action=”submit” method=”get”>
<input class=”username” type=”text” />
<input class=”password” type=”password” />
</form>
element = driver.find_element_by_css_selector(“form#testform>input.username”)
Important Modules to Import:
from selenium import webdriver
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.ui import Select
from selenium.webdriver.common.by import By
from selenium.webdriver.common.action_chains import ActionChains
Python 3 Cheat Sheet
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.firefox.firefox_binary import FirefoxBinary
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.firefox.options import Options
Python Selenium commands for operation on elements:
button/link/image:
click()
get_attribute()
is_displayed()
is_enabled()
Text field:
send_keys()
clear()
Checkbox/Radio:
is_selected()
click()
Select:
Find out the select element using any element locating strategies and then select options from list using index, visible text or option value.
Element properties:
is_displayed()
is_selected()
is_enabled()
These methods return either true or false.
Read Attribute:
get_attribute(“”)
Get attribute from a disabled text box
driver.find_element_by_id(“id”).get_attribute(“value”);
Screenshot:
Note: An important note to store screenshots is that save_screenshot(‘filename’) and get_screenshot_as_file(‘filename’) will work only when extension of file is ‘.png’. Otherwise content of the screenshot can’t be viewed
Read articles for more details about taking screenshot and element screenshot
The list here contains mostly used python selenium commands but not exhaustive. Please feel free to add in comments if you feel something is missing and should be here.
3 Responses
[…] Previous: Previous post: Execute Python Selenium tests in Selenium GridNext: Next post: Python selenium commands cheat sheet […]
Thank you very much
Hi Sir,
I am trying to do the sorting in selenium with python using For loop could u please help me is there any way that i can do it ?
Sorting in descending order in the below website
website: https://jqueryui.com/sortable/
Python Basics Cheat Sheet
Python is one of the most popular programming languages in networking World. Almost all network engineers learn and use this programming language in their daily works. Because of the fact that there are many details in Python as in all programming languages, sometimes we can forget a basic command or a general concept, usage. Python Cheat Sheet has created to overcome this case and aims to remind you the missing points of this awesome network programming language. It is like other cheat sheets like CLI Command Cheat Sheet, Linux Commands Cheat Sheetetc.
You can use Python in another area than networking certainly. Whichever you use, the concepts and usage of the programming language are similar. There are only small differences and focus change in classical programming and network programming and automation. This Python Cheat Sheet will help you not only on your network automation or network programming activities, but also it will help you in all your programming works even in another area than computer networking. So, this page will be a reference page both programmers or network engineers. Because both of these jobs use Python and the concepts sof this programming language is similar.
There are many Python tutorial on internet and you can download Python free on internet. But it is difficult to have such a Ptyhon Cheat Sheet, that covers almost all important parts of Ptyhon programming language. It can be a quick reference for you or a document that you remind key parts. Whichever it is, this page will help you a lot and will decrease your exploration period for any code part.
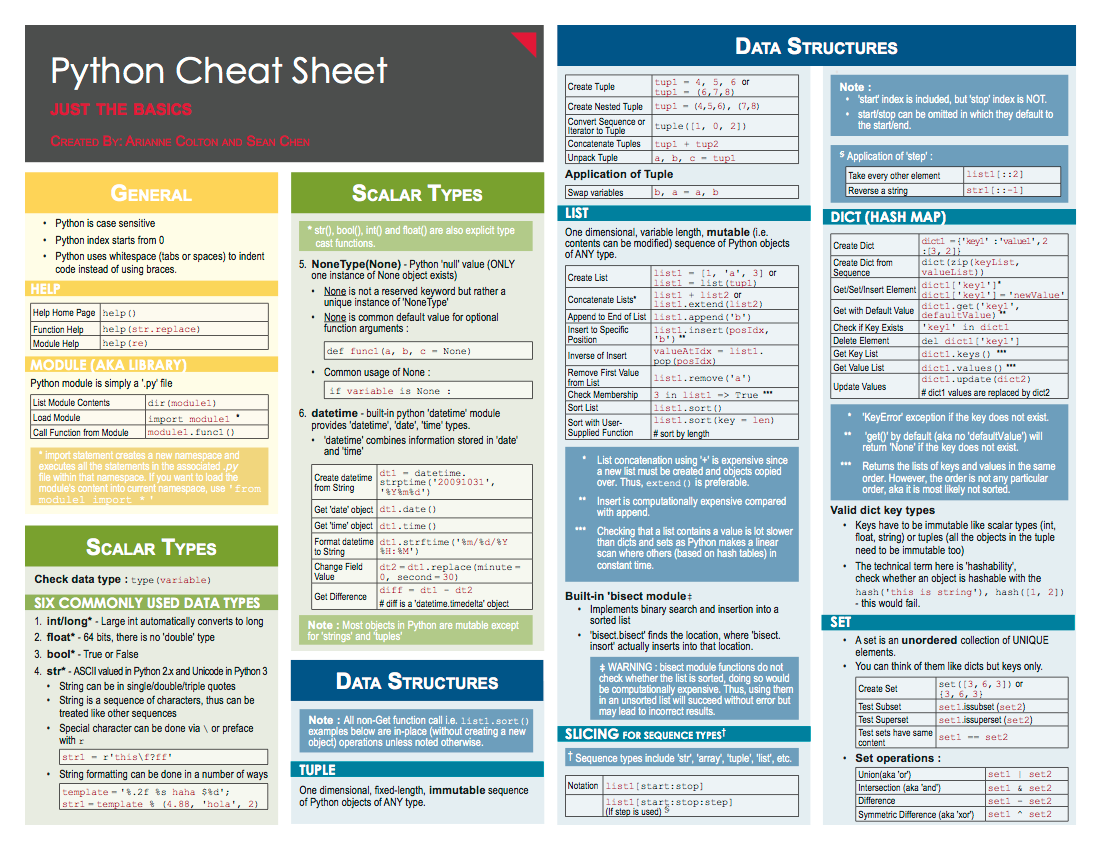
You can find Python list, Python range, Python class, Python dictionary or any other concepts on this page. We will cover all basic Python terms here. So, by having Pyhton Cheat Sheet, you will have a strong partner with you during your Ptyhon adventure.
Python Cheat Sheet For Beginners
Python Cheat Sheet has prepared for both beginner users and Python experts. So, you can use this cheat sheet as Pyhton Cheat Sheet For Beginners and For Experts. The programming language is similar and in this page, we will cover all these basic concepts.
If you use this sheet as Python beginner cheat sheet, you can use it during your programming activities. You can download this cheat sheet and you can use it on your computer during code writing. You can also use this Ptyhon Cheat Sheet online.
If you use this sheet as expert reference, you can use it whenever you need to remember a Python code or usage. This page will help you in your critical coding activities.
Every tech guy was a beginner before. So, if you are a beginner now, you will be an expert too in the future. During this period, during your Python journey, this document will be always with you and you will benefit a lot from this page.
Python Cheat Sheet Pdf
This reference document can be used both online on our website or you can download Python Cheat Sheetpdf and use offline on your computer. Whichever you use, this excellent Ptyhon Cheat Sheet pdf will help you a lot.
When you downloadPython Cheat Sheet Pdf, you can use it to remember any Python code. This can be any Python code. Maybe you do not remember, Python list, Python dictionary, Python ranges etc. Maybe you remember the codes but you forgot the usage. Whichever it is, you can find on this page and with this page, you will not struggle on internet to find any Python code.
Python Interview Cheat Sheet
Python Cheat Sheet can be used also for your Python Job interview. Before your technical interview, you can check this sheet and you can use it as Python Interview Cheat Sheet. You can find all the basic terms of Python programming languages in this cheat sheet. So, the questions in you technical Python interview will be mostly on this Python Interview Cheat Sheet.
In your Python interview, maybe they will ask how to use Python tubles? Maybe they want to learn, how to get the last term in a Python list. Or maybe their question will be, how to get different types of inputs from the user.
Python Cheat Sheet Download
Beside basic questions, in the Python Interview, they can ask complex questions about a programming code part. They can ask any specific parts of a Python program in the interview. Python Interview Cheat Sheet can remind you basic terms for this complex questions.
If You Like, Kindly Share
If you find this page useful for your Python works and if you like it, kindly share this page with your friends, with your colleagues, with your social media followers etc. Whoever you share this knowledge, this will help us to develop better cheat sheets.
You can share this page in your social media accounts like facebook, linkedin, twitter etc., in related network and programming forums, in your blogs, in any of your accounts. If you share this page, this page will help another network, programming fan and it eases his/her work. So, if you would like to help others, kindly share this page.
Python Functions Cheat Sheet
Do not forget, Knowledge Increases by Sharing.
